Last Updated on October 6, 2023 by KnownSense
In this article we’re going to look at the API gateways which are commonly used within microservices architecture to provide a central entry point for our client applications. API gateway is normally an off‑the‑shelf product or a service provided on a cloud platform, it basically provides one interface to our back‑end microservices for our client applications. This basically means our client applications only need to remember the API gateway for the data and the functionalities they need, and therefore, they only need to remember the location of one component instead of having to remember the location of every single microservice in the background that they might eventually need. The API gateway basically provides a number of endpoints to our client applications, and it takes incoming requests on those endpoints and then satisfies those requests by calling our downstream microservices for specific data and functionality. All this mapping between incoming requests and downstream microservices is done using configuration within the API gateway.
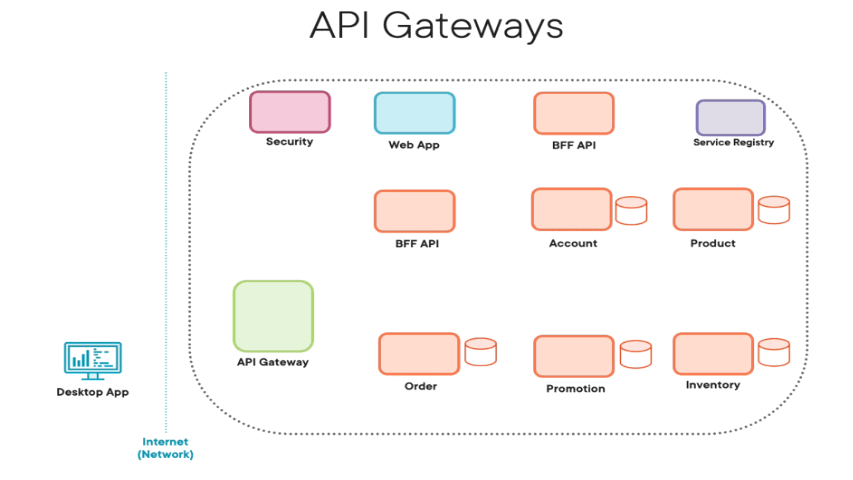
Benefits
- Centralized API Management: An API Gateway serves as a centralized point for managing and routing API requests. It provides a single entry point for clients to access various microservices or backend services, simplifying the client experience.
- Request Routing: The API gateway is responsible for routing client requests to the appropriate microservice based on the requested URL or path. It acts as a reverse proxy, forwarding requests to the relevant service.
- Security: API Gateways can enforce security measures such as authentication, authorization, rate limiting, and API key management. This helps protect your backend services from unauthorized access and abuse.
- Load Balancing: API Gateways distribute incoming requests across multiple instances of a service, ensuring high availability and optimal resource utilization. They can also handle failover when backend services become unavailable.
- Rate-limiting: API gateways can also provide services like call rate limiting to control the number of incoming requests.
- Logging and Monitoring: API Gateways can collect valuable metrics, logs, and analytics data about API usage. This information is essential for troubleshooting, performance optimization, and auditing.
- Transformation and Aggregation: API Gateways can transform and aggregate responses from multiple microservices, providing clients with a unified and tailored API. This reduces the need for clients to make multiple requests to different services.
- Caching: API Gateways can cache responses, reducing the load on backend services and improving response times for frequently accessed data.
- Cross-cutting Concerns: They handle cross-cutting concerns like request/response logging, request/response compression, and protocol translation, allowing backend services to focus on their core functionality.
- Versioning: API Gateways can handle API versioning, making it easier to introduce breaking changes to APIs without affecting existing clients.
- Protocol Bridging: When microservices use different communication protocols or data formats, the API gateway can act as a bridge, translating between them.
- Rate Limiting: To prevent abuse, the API Gateway enforces rate limits. It allows a limited number of requests per minute for each user.
- SSL Termination: In a microservices architecture, an API gateway is a server that acts as an entry point for all incoming client requests. It serves as a central point of control and coordination for managing and routing requests to various microservices within the system.
- Error Handling: They handle errors gracefully, providing meaningful error messages to clients and logging detailed error information for debugging purposes.
E-commerce platform with gateway
Let’s consider an e-commerce platform as an example and will read the benefits it get from gateway
- Authentication and Authorization: The API Gateway handles user authentication using OAuth 2.0. It verifies user credentials and issues access tokens. It also enforces role-based access control, ensuring that only authorized users can access certain endpoints.
- Load Balancing: When a user searches for a product, the API Gateway routes the request to one of several search service instances. It uses load balancing algorithms to distribute traffic evenly.
- Caching: Product details, such as descriptions and images, are cached by the API Gateway. This reduces the load on the product information service and improves response times for frequently viewed products.
- Versioning: The API Gateway supports API versioning. It can route requests to different versions of the product service based on the requested API version.
- Request Aggregation: When a user views a product, the API Gateway fetches data from multiple services (e.g., product information, reviews, inventory). It aggregates this data and sends a single response to the client, simplifying the client’s experience.
- Logging and Monitoring: The API Gateway logs API requests and responses, providing valuable insights into user behavior and service performance. It integrates with a monitoring solution to track the health of backend services.
By using an API Gateway, the e-commerce platform achieves a more secure, scalable, and efficient API ecosystem while simplifying the development experience for client applications. It centralizes various concerns and provides a unified interface for clients to interact with the underlying microservices.
Spring Cloud Gateway (SCG): A Dynamic Gateway Solution
SCG is an open-source, non-blocking, and reactive API gateway built on Spring WebFlux. It provides essential functionalities for routing, filtering, and managing HTTP requests in microservices-based applications.
Below are the steps with code snippets for creating a basic Spring Cloud Gateway application:
Step 1: Create a Spring Boot Project
Use Spring Initializr or your preferred IDE to create a new Spring Boot project with the necessary dependencies, including “Spring Cloud Gateway.”
Step 2: Define Route Configuration
In your project’s application.yml
(or application.properties
), define route configurations to specify how requests should be routed to backend services:
spring:
cloud:
gateway:
routes:
- id: service-route
uri: http://backend-service:8080
predicates:
- Path=/api/**
In this example, requests with a path starting with /api/
are routed to the backend-service
running on port 8080.
Step 3: Create a Spring Cloud Gateway Application
Create a main class for your Spring Cloud Gateway application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
Step 4: Implement Filters (Optional)
You can create custom filters to modify or enhance requests and responses. Here’s an example of a custom filter that logs incoming requests:
import org.springframework.cloud.gateway.filter.GlobalFilter;
import org.springframework.core.Ordered;
import org.springframework.stereotype.Component;
import reactor.core.publisher.Mono;
@Component
public class LoggingFilter implements GlobalFilter, Ordered {
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
// Implement your logging logic here
return chain.filter(exchange);
}
@Override
public int getOrder() {
return 0; // Filter order (modify as needed)
}
}
Step 5: Run and Test
Start your Spring Cloud Gateway application, and it will listen on a specified port (default is 8080). Send HTTP requests to the gateway and observe how they are routed based on the route configuration.
Step 6: Scaling and Production Deployment
As your application grows, consider scaling the Spring Cloud Gateway instances to handle increased traffic efficiently. Deploy the gateway as part of your microservices architecture in a production environment.
These are the basic steps to create a Spring Cloud Gateway application. You can further enhance your gateway with additional features like authentication, rate limiting, and more complex routing configurations based on your specific requirements.
Conclusion
API gateway, exemplified by Spring Cloud Gateway, have become essential components in building robust, secure, and efficient microservices architectures. They act as gatekeepers, enabling organizations to effectively manage the complex interactions between clients and services while offering a range of features that enhance security, scalability, and developer productivity. Embracing the capabilities of API gateways is a strategic choice for organizations looking to build and maintain resilient and high-performing distributed systems.